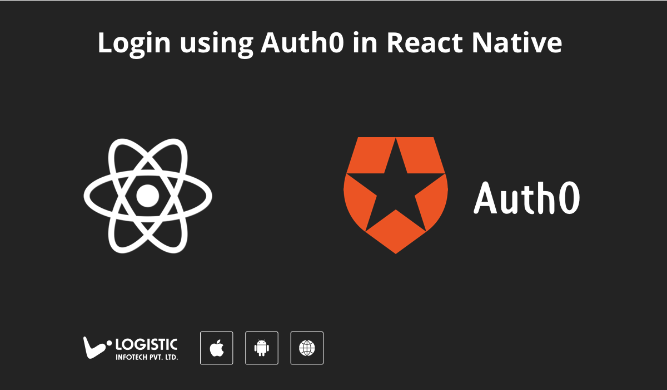
For any great product to be successful they must have to connect with social media. Social networks encourage us to add a social dimension in our mobile application. Often it can be social authentication or a content share with social media like Facebook, LinkedIn, Google, Twitter etc… Nowadays most of the applications allow you to do social authentication and share content through them. With social media, the login process become easier and smoother.
Social Authentication using Auth0
Most social networks offers their own native SDK, for each social integration. You might have to use different SDKs for each social platform is not a preferable way. You might wish that single SDK provide a universal authentication & authorization platform for your application.
So here in this article, you will learn how to integrate different social networks with a single SDK which is Auth0. Auth0 provide a universal authentication & authorization platform for web, mobile, and legacy applications. In this article, you will learn how to integrate Auth0 with your React Native application.
Install Dependencies
For Auth0 integration, they provide their own npm package, it’ s very easy to integrate with your app. You need to run below command to add Auth0 package.
npm install react-native-auth0 —save
To use the package you need to link with your React application using below command.
react-native link react-native-auth0
Configure for iOS
For this, you need to open the Xcode project from your iOS folder of your React Native project. Add the following method in your AppDelegate.m file as given below.
- (BOOL)application:(UIApplication *)application openURL:(NSURL *)url sourceApplication:(NSString *)sourceApplication annotation:(id)annotation { return [RCTLinkingManager application:application openURL:url sourceApplication:sourceApplication annotation:annotation]; }
Now you need to set up URLScheme in your Info.plist file. Go to Info.plist and locate the value for CFBundleIdentifier of your application as written below.
<key>CFBundleIdentifier</key> <string>com.yourcompany.$(PRODUCT_NAME:rfc1034identifier)</string>
Then register a URL type entry using the value of CFBundleIdentifier as the value for the CFBundleURLSchemes.
<key>CFBundleURLTypes</key> <array> <dict> <key>CFBundleTypeRole</key> <string>None</string> <key>CFBundleURLName</key> <string>auth0</string> <key>CFBundleURLSchemes</key> <array> <string>com.yourcompany.$(PRODUCT_NAME:rfc1034identifier)</string> </array> </dict> </array>
Configure for Android
You need to place below code snippets in your AndroidManifest.xml file like below.
<activity android:name=".MainActivity" ...> <intent-filter> <action android:name="android.intent.action.VIEW" /> <category android:name="android.intent.category.DEFAULT" /> <category android:name="android.intent.category.BROWSABLE" /> <data android:host="logistic.auth0.com" android:pathPrefix="/android/${applicationId}/callback" android:scheme="${applicationId}" /> </intent-filter> </activity>
Authentication with Auth0 using React Native
Let’s import Auth0 module in your React Native application and create a new Auth0 instance as shown in below code.
import Auth0 from 'react-native-auth0'; const auth0 = new Auth0({ domain: 'your auth0 domain', clientId: 'your auth0 clientId' });
To get your auth0 domain and clientId you need to register with auth0.
Here is the code snippet to login with Google+ in your application.
auth0.webAuth .authorize({ scope: 'openid profile email', audience: 'https://logisticinfotech.auth0.com/userinfo', connection: 'google-oauth2', // }) .then( credentials => { console.log('Auth0 Credential :==> \n', JSON.stringify(credentials)); let idToken = credentials.accessToken; auth0.auth .userInfo({ token: idToken }) .then(userData => { console.log('User Data From Auth0 :===> \n', JSON.stringify(userData.name)); }) .catch(console.error); } ) .catch(error => { console.log('Auth0 Error :==> \n', error); });
This is a simple API call we normally use. In that you need to add authorized social network like Google, Facebook etc.. as a connection and it will be redirected to that social network login page. After that API call, you get account information of that particular user and access token in a JSON response. If there is any problem in authentication then it will display an error otherwise it will login successfully in your app and will save the user data.
Same for the other social account login you just need to change connection type and you will integrate the other social network in your application.
// For facebook authorize({ audience: 'https://logisticinfotech.auth0.com/userinfo', connection: 'facebook', }) // For linkedIn authorize({ audience: 'https://logisticinfotech.auth0.com/userinfo', connection: 'linkedin', })
Likewise, you can integrate authentication using a single SDK of Auth0.
Implement logout with Auth0
Once you are loggedIn with social network and it may be necessary to allow the user to logout and login via another social network. For that, you may need to clear a previous social network using the logout option. Let’s understand with code snippet given below.
auth0.webAuth .clearSession() .then(res => { console.log("error clearing session: ", err); }) .catch(err => { console.log("error clearing session: ", err); });
Its simple API call similar to authentication call written above for social login. Once its executed it will clear all session in the network and you might be able to login via another social account you want.
Finally, you may have learned how easily you can integrate social login without any headache of implementation of different different APIs for each social platform you are going to use. Auth0 provide a very robust and easy way to integrate social login with your application. There are many other features provided by Auth0 other than social login. You may learn those in our next articles.